진짜 비효율적으로 삽하면서 풀어놓고 조금 현타옴
문제
You are given an integer array 가격 where prices(i) is the price of a given stock on the ith day.
On each day, you may decide to buy and/or sell the stock. You can only hold at most one share of the stock at any time. However, you can buy it then immediately sell it on the same day.
Find and return 더 maximum profit you can achieve.
정수 배열 가격이 주어집니다.
여기서 가격(i)은 해당 날짜의 주가입니다.
매일 주식을 구매 및/또는 판매할 수 있습니다.
한 번에 최대 1종의 주식만 보유할 수 있습니다.
그러나 구입 후 같은 날 빨리 팔 수 있습니다.
달성할 수 있는 최대 수익를 찾아 반환합니다.
예
예 1:
Input: prices = (7,1,5,3,6,4)
Output: 7
Explanation: Buy on day 2 (price = 1) and sell on day 3 (price = 5), profit = 5-1 = 4.
Then buy on day 4 (price = 3) and sell on day 5 (price = 6), profit = 6-3 = 3.
Total profit is 4 + 3 = 7.
예 2:
Input: prices = (1,2,3,4,5)
Output: 4
Explanation: Buy on day 1 (price = 1) and sell on day 5 (price = 5), profit = 5-1 = 4.
Total profit is 4.
예 3:
Input: prices = (7,6,4,3,1)
Output: 0
Explanation: There is no way to make a positive profit, so we never buy the stock to achieve the maximum profit of 0.
해결 코드
var maxProfit = function(prices) {
let start = 0;
let end = 0;
let sum = 0;
for (let i = 0; i<prices.length-1; i++) {
if(prices(i) >= prices(i+1)) {
if(start < end) {sum+= prices(end) - prices(start)}
start = i+1;
} else {
end = i+1;
}
if(i === prices.length-2 && start < end) {
sum += prices(end) - prices(start)
}
}
return sum;
};
생각하다
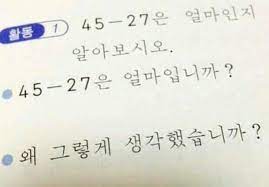
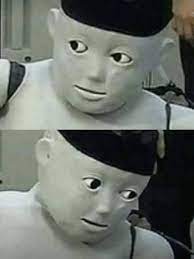
(1,2,3) 이러한 경우에 최대 프로피트를 요구하는 것
(2-1)+(3-2)와 3-1이 같다는 것을… 왜 상기할 수 없었는가? 뒤에 큰 숫자가 오면, 단지 기분 좋게 당겨 주면 좋다.
start까지는 필요 없었다는 것이다!
!
!
!
이번에는 너무 중복으로 풀어 조금 심란했다.
보완
var maxProfit = function(prices) {
let profit = 0
for(let i=0; i < prices.length; i++) {
if(prices(i) > prices(i-1)) {
profit += prices(i) - prices(i-1);
}
}
return profit;
};
제대로 무난한 방법이 있습니다.
i+1 하면, 마지막 요소를 순회할 때에 배열장보다 긴 인덱스를 찾으려고 하기 때문에, i-1 하는 것이 좋다